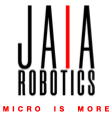 |
JaiaBot
1.19.0
JaiaBot micro-AUV software
|
|
#include <jaiabot/messages/health.pb.h>
Definition at line 1842 of file health.pb.h.
◆ LeapIndicator
◆ NTPPeer
◆ NTPSystemEvent
◆ SyncSource
◆ NTPStatus() [1/2]
jaiabot::protobuf::NTPStatus::NTPStatus |
( |
| ) |
|
◆ ~NTPStatus()
virtual jaiabot::protobuf::NTPStatus::~NTPStatus |
( |
| ) |
|
|
virtual |
◆ NTPStatus() [2/2]
jaiabot::protobuf::NTPStatus::NTPStatus |
( |
const NTPStatus & |
from | ) |
|
◆ add_peer()
◆ ByteSizeLong()
size_t jaiabot::protobuf::NTPStatus::ByteSizeLong |
( |
| ) |
const |
|
final |
◆ Clear()
void jaiabot::protobuf::NTPStatus::Clear |
( |
| ) |
|
|
final |
◆ clear_last_system_event()
void jaiabot::protobuf::NTPStatus::clear_last_system_event |
( |
| ) |
|
|
inline |
◆ clear_leap_indicator()
void jaiabot::protobuf::NTPStatus::clear_leap_indicator |
( |
| ) |
|
|
inline |
◆ clear_peer()
void jaiabot::protobuf::NTPStatus::clear_peer |
( |
| ) |
|
|
inline |
◆ clear_sync_source()
void jaiabot::protobuf::NTPStatus::clear_sync_source |
( |
| ) |
|
|
inline |
◆ clear_system_event_counter()
void jaiabot::protobuf::NTPStatus::clear_system_event_counter |
( |
| ) |
|
|
inline |
◆ clear_system_sync_peer()
void jaiabot::protobuf::NTPStatus::clear_system_sync_peer |
( |
| ) |
|
|
inline |
◆ CopyFrom() [1/2]
void jaiabot::protobuf::NTPStatus::CopyFrom |
( |
const ::google::protobuf::Message & |
from | ) |
|
|
final |
◆ CopyFrom() [2/2]
void jaiabot::protobuf::NTPStatus::CopyFrom |
( |
const NTPStatus & |
from | ) |
|
◆ default_instance()
static const NTPStatus& jaiabot::protobuf::NTPStatus::default_instance |
( |
| ) |
|
|
static |
◆ descriptor()
static const ::google::protobuf::Descriptor* jaiabot::protobuf::NTPStatus::descriptor |
( |
| ) |
|
|
static |
◆ GetCachedSize()
int jaiabot::protobuf::NTPStatus::GetCachedSize |
( |
| ) |
const |
|
inlinefinal |
◆ GetMetadata()
::google::protobuf::Metadata jaiabot::protobuf::NTPStatus::GetMetadata |
( |
| ) |
const |
|
final |
◆ has_last_system_event()
bool jaiabot::protobuf::NTPStatus::has_last_system_event |
( |
| ) |
const |
|
inline |
◆ has_leap_indicator()
bool jaiabot::protobuf::NTPStatus::has_leap_indicator |
( |
| ) |
const |
|
inline |
◆ has_sync_source()
bool jaiabot::protobuf::NTPStatus::has_sync_source |
( |
| ) |
const |
|
inline |
◆ has_system_event_counter()
bool jaiabot::protobuf::NTPStatus::has_system_event_counter |
( |
| ) |
const |
|
inline |
◆ has_system_sync_peer()
bool jaiabot::protobuf::NTPStatus::has_system_sync_peer |
( |
| ) |
const |
|
inline |
◆ InitAsDefaultInstance()
static void jaiabot::protobuf::NTPStatus::InitAsDefaultInstance |
( |
| ) |
|
|
static |
◆ internal_default_instance()
static const NTPStatus* jaiabot::protobuf::NTPStatus::internal_default_instance |
( |
| ) |
|
|
inlinestatic |
◆ InternalSerializeWithCachedSizesToArray()
::google::protobuf::uint8* jaiabot::protobuf::NTPStatus::InternalSerializeWithCachedSizesToArray |
( |
bool |
deterministic, |
|
|
::google::protobuf::uint8 * |
target |
|
) |
| const |
|
final |
◆ IsInitialized()
bool jaiabot::protobuf::NTPStatus::IsInitialized |
( |
| ) |
const |
|
final |
◆ last_system_event()
◆ leap_indicator()
◆ LeapIndicator_descriptor()
static const ::google::protobuf::EnumDescriptor* jaiabot::protobuf::NTPStatus::LeapIndicator_descriptor |
( |
| ) |
|
|
inlinestatic |
◆ LeapIndicator_IsValid()
static bool jaiabot::protobuf::NTPStatus::LeapIndicator_IsValid |
( |
int |
value | ) |
|
|
inlinestatic |
◆ LeapIndicator_Name()
static const ::std::string& jaiabot::protobuf::NTPStatus::LeapIndicator_Name |
( |
LeapIndicator |
value | ) |
|
|
inlinestatic |
◆ LeapIndicator_Parse()
static bool jaiabot::protobuf::NTPStatus::LeapIndicator_Parse |
( |
const ::std::string & |
name, |
|
|
LeapIndicator * |
value |
|
) |
| |
|
inlinestatic |
◆ MergeFrom() [1/2]
void jaiabot::protobuf::NTPStatus::MergeFrom |
( |
const ::google::protobuf::Message & |
from | ) |
|
|
final |
◆ MergeFrom() [2/2]
void jaiabot::protobuf::NTPStatus::MergeFrom |
( |
const NTPStatus & |
from | ) |
|
◆ MergePartialFromCodedStream()
bool jaiabot::protobuf::NTPStatus::MergePartialFromCodedStream |
( |
::google::protobuf::io::CodedInputStream * |
input | ) |
|
|
final |
◆ mutable_peer() [1/2]
◆ mutable_peer() [2/2]
◆ mutable_system_sync_peer()
◆ mutable_unknown_fields()
inline ::google::protobuf::UnknownFieldSet* jaiabot::protobuf::NTPStatus::mutable_unknown_fields |
( |
| ) |
|
|
inline |
◆ New() [1/2]
NTPStatus* jaiabot::protobuf::NTPStatus::New |
( |
| ) |
const |
|
inlinefinal |
◆ New() [2/2]
NTPStatus* jaiabot::protobuf::NTPStatus::New |
( |
::google::protobuf::Arena * |
arena | ) |
const |
|
inlinefinal |
◆ NTPSystemEvent_descriptor()
static const ::google::protobuf::EnumDescriptor* jaiabot::protobuf::NTPStatus::NTPSystemEvent_descriptor |
( |
| ) |
|
|
inlinestatic |
◆ NTPSystemEvent_IsValid()
static bool jaiabot::protobuf::NTPStatus::NTPSystemEvent_IsValid |
( |
int |
value | ) |
|
|
inlinestatic |
◆ NTPSystemEvent_Name()
static const ::std::string& jaiabot::protobuf::NTPStatus::NTPSystemEvent_Name |
( |
NTPSystemEvent |
value | ) |
|
|
inlinestatic |
◆ NTPSystemEvent_Parse()
static bool jaiabot::protobuf::NTPStatus::NTPSystemEvent_Parse |
( |
const ::std::string & |
name, |
|
|
NTPSystemEvent * |
value |
|
) |
| |
|
inlinestatic |
◆ operator=()
◆ peer() [1/2]
◆ peer() [2/2]
◆ peer_size()
int jaiabot::protobuf::NTPStatus::peer_size |
( |
| ) |
const |
|
inline |
◆ release_system_sync_peer()
◆ SerializeWithCachedSizes()
void jaiabot::protobuf::NTPStatus::SerializeWithCachedSizes |
( |
::google::protobuf::io::CodedOutputStream * |
output | ) |
const |
|
final |
◆ set_allocated_system_sync_peer()
◆ set_last_system_event()
◆ set_leap_indicator()
◆ set_sync_source()
◆ set_system_event_counter()
void jaiabot::protobuf::NTPStatus::set_system_event_counter |
( |
::google::protobuf::int32 |
value | ) |
|
|
inline |
◆ Swap()
void jaiabot::protobuf::NTPStatus::Swap |
( |
NTPStatus * |
other | ) |
|
◆ sync_source()
◆ SyncSource_descriptor()
static const ::google::protobuf::EnumDescriptor* jaiabot::protobuf::NTPStatus::SyncSource_descriptor |
( |
| ) |
|
|
inlinestatic |
◆ SyncSource_IsValid()
static bool jaiabot::protobuf::NTPStatus::SyncSource_IsValid |
( |
int |
value | ) |
|
|
inlinestatic |
◆ SyncSource_Name()
static const ::std::string& jaiabot::protobuf::NTPStatus::SyncSource_Name |
( |
SyncSource |
value | ) |
|
|
inlinestatic |
◆ SyncSource_Parse()
static bool jaiabot::protobuf::NTPStatus::SyncSource_Parse |
( |
const ::std::string & |
name, |
|
|
SyncSource * |
value |
|
) |
| |
|
inlinestatic |
◆ system_event_counter()
google::protobuf::int32 jaiabot::protobuf::NTPStatus::system_event_counter |
( |
| ) |
const |
|
inline |
◆ system_sync_peer()
◆ unknown_fields()
const ::google::protobuf::UnknownFieldSet& jaiabot::protobuf::NTPStatus::unknown_fields |
( |
| ) |
const |
|
inline |
◆ ::protobuf_jaiabot_2fmessages_2fhealth_2eproto::TableStruct
◆ swap
◆ kIndexInFileMessages
constexpr int jaiabot::protobuf::NTPStatus::kIndexInFileMessages |
|
staticconstexpr |
◆ kLastSystemEventFieldNumber
const int jaiabot::protobuf::NTPStatus::kLastSystemEventFieldNumber = 13 |
|
static |
◆ kLeapIndicatorFieldNumber
const int jaiabot::protobuf::NTPStatus::kLeapIndicatorFieldNumber = 11 |
|
static |
◆ kPeerFieldNumber
const int jaiabot::protobuf::NTPStatus::kPeerFieldNumber = 21 |
|
static |
◆ kSyncSourceFieldNumber
const int jaiabot::protobuf::NTPStatus::kSyncSourceFieldNumber = 10 |
|
static |
◆ kSystemEventCounterFieldNumber
const int jaiabot::protobuf::NTPStatus::kSystemEventCounterFieldNumber = 12 |
|
static |
◆ kSystemSyncPeerFieldNumber
const int jaiabot::protobuf::NTPStatus::kSystemSyncPeerFieldNumber = 20 |
|
static |
◆ LEAP_CLOCK_NOT_SYNCHRONIZED
const LeapIndicator jaiabot::protobuf::NTPStatus::LEAP_CLOCK_NOT_SYNCHRONIZED |
|
static |
◆ LEAP_LAST_MINUTE_HAS_59_SECONDS
const LeapIndicator jaiabot::protobuf::NTPStatus::LEAP_LAST_MINUTE_HAS_59_SECONDS |
|
static |
◆ LEAP_LAST_MINUTE_HAS_61_SECONDS
const LeapIndicator jaiabot::protobuf::NTPStatus::LEAP_LAST_MINUTE_HAS_61_SECONDS |
|
static |
◆ LEAP_NONE
◆ LEAP_UNKNOWN
◆ LeapIndicator_ARRAYSIZE
const int jaiabot::protobuf::NTPStatus::LeapIndicator_ARRAYSIZE |
|
static |
◆ LeapIndicator_MAX
const LeapIndicator jaiabot::protobuf::NTPStatus::LeapIndicator_MAX |
|
static |
◆ LeapIndicator_MIN
const LeapIndicator jaiabot::protobuf::NTPStatus::LeapIndicator_MIN |
|
static |
◆ NTP_SYSTEM_CLOCK_STEP
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_CLOCK_STEP |
|
static |
◆ NTP_SYSTEM_CLOCK_SYNC
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_CLOCK_SYNC |
|
static |
◆ NTP_SYSTEM_EVENT_UNKNOWN
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_EVENT_UNKNOWN |
|
static |
◆ NTP_SYSTEM_EVENT_UNSPECIFIED
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_EVENT_UNSPECIFIED |
|
static |
◆ NTP_SYSTEM_FREQ_MODE
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_FREQ_MODE |
|
static |
◆ NTP_SYSTEM_FREQ_NOT_SET
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_FREQ_NOT_SET |
|
static |
◆ NTP_SYSTEM_FREQ_SET
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_FREQ_SET |
|
static |
◆ NTP_SYSTEM_KERNEL_INFO
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_KERNEL_INFO |
|
static |
◆ NTP_SYSTEM_LEAP_ARMED
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_LEAP_ARMED |
|
static |
◆ NTP_SYSTEM_LEAP_DISARMED
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_LEAP_DISARMED |
|
static |
◆ NTP_SYSTEM_LEAP_EVENT
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_LEAP_EVENT |
|
static |
◆ NTP_SYSTEM_LEAPSECOND_VALUES_UPDATE_FROM_FILE
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_LEAPSECOND_VALUES_UPDATE_FROM_FILE |
|
static |
◆ NTP_SYSTEM_NO_SYSTEM_PEER
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_NO_SYSTEM_PEER |
|
static |
◆ NTP_SYSTEM_PANIC_STOP
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_PANIC_STOP |
|
static |
◆ NTP_SYSTEM_RESTART
◆ NTP_SYSTEM_SPIKE_DETECT
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_SPIKE_DETECT |
|
static |
◆ NTP_SYSTEM_STALE_LEAPSECOND_VALUES
const NTPSystemEvent jaiabot::protobuf::NTPStatus::NTP_SYSTEM_STALE_LEAPSECOND_VALUES |
|
static |
◆ NTPSystemEvent_ARRAYSIZE
const int jaiabot::protobuf::NTPStatus::NTPSystemEvent_ARRAYSIZE |
|
static |
◆ NTPSystemEvent_MAX
◆ NTPSystemEvent_MIN
◆ SYNC_HF_RADIO
const SyncSource jaiabot::protobuf::NTPStatus::SYNC_HF_RADIO |
|
static |
◆ SYNC_LF_RADIO
const SyncSource jaiabot::protobuf::NTPStatus::SYNC_LF_RADIO |
|
static |
◆ SYNC_LOCAL
const SyncSource jaiabot::protobuf::NTPStatus::SYNC_LOCAL |
|
static |
◆ SYNC_NTP
const SyncSource jaiabot::protobuf::NTPStatus::SYNC_NTP |
|
static |
◆ SYNC_OTHER
const SyncSource jaiabot::protobuf::NTPStatus::SYNC_OTHER |
|
static |
◆ SYNC_PPS
const SyncSource jaiabot::protobuf::NTPStatus::SYNC_PPS |
|
static |
◆ SYNC_TELEPHONE
const SyncSource jaiabot::protobuf::NTPStatus::SYNC_TELEPHONE |
|
static |
◆ SYNC_UHF_RADIO
const SyncSource jaiabot::protobuf::NTPStatus::SYNC_UHF_RADIO |
|
static |
◆ SYNC_UNKNOWN
const SyncSource jaiabot::protobuf::NTPStatus::SYNC_UNKNOWN |
|
static |
◆ SYNC_UNSPECIFIED
const SyncSource jaiabot::protobuf::NTPStatus::SYNC_UNSPECIFIED |
|
static |
◆ SYNC_WRISTWATCH
const SyncSource jaiabot::protobuf::NTPStatus::SYNC_WRISTWATCH |
|
static |
◆ SyncSource_ARRAYSIZE
const int jaiabot::protobuf::NTPStatus::SyncSource_ARRAYSIZE |
|
static |
◆ SyncSource_MAX
const SyncSource jaiabot::protobuf::NTPStatus::SyncSource_MAX |
|
static |
◆ SyncSource_MIN
const SyncSource jaiabot::protobuf::NTPStatus::SyncSource_MIN |
|
static |
The documentation for this class was generated from the following file:
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_FREQ_MODE
@ NTPStatus_SyncSource_SYNC_LF_RADIO
@ NTPStatus_SyncSource_SYNC_HF_RADIO
const NTPStatus_NTPSystemEvent NTPStatus_NTPSystemEvent_NTPSystemEvent_MAX
@ NTPStatus_SyncSource_SYNC_PPS
@ NTPStatus_SyncSource_SYNC_OTHER
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_LEAPSECOND_VALUES_UPDATE_FROM_FILE
const NTPStatus_NTPSystemEvent NTPStatus_NTPSystemEvent_NTPSystemEvent_MIN
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_LEAP_ARMED
const NTPStatus_LeapIndicator NTPStatus_LeapIndicator_LeapIndicator_MAX
@ NTPStatus_LeapIndicator_LEAP_LAST_MINUTE_HAS_61_SECONDS
@ NTPStatus_LeapIndicator_LEAP_NONE
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_NO_SYSTEM_PEER
const NTPStatus_SyncSource NTPStatus_SyncSource_SyncSource_MIN
@ NTPStatus_LeapIndicator_LEAP_UNKNOWN
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_LEAP_EVENT
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_EVENT_UNSPECIFIED
const int NTPStatus_LeapIndicator_LeapIndicator_ARRAYSIZE
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_SPIKE_DETECT
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_STALE_LEAPSECOND_VALUES
@ NTPStatus_SyncSource_SYNC_LOCAL
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_LEAP_DISARMED
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_FREQ_SET
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_KERNEL_INFO
const int NTPStatus_SyncSource_SyncSource_ARRAYSIZE
@ NTPStatus_SyncSource_SYNC_WRISTWATCH
@ NTPStatus_LeapIndicator_LEAP_LAST_MINUTE_HAS_59_SECONDS
@ NTPStatus_SyncSource_SYNC_UNSPECIFIED
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_PANIC_STOP
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_RESTART
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_CLOCK_SYNC
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_CLOCK_STEP
const NTPStatus_SyncSource NTPStatus_SyncSource_SyncSource_MAX
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_EVENT_UNKNOWN
@ NTPStatus_NTPSystemEvent_NTP_SYSTEM_FREQ_NOT_SET
@ NTPStatus_SyncSource_SYNC_NTP
const NTPStatus_LeapIndicator NTPStatus_LeapIndicator_LeapIndicator_MIN
@ NTPStatus_SyncSource_SYNC_UHF_RADIO
@ NTPStatus_LeapIndicator_LEAP_CLOCK_NOT_SYNCHRONIZED
const int NTPStatus_NTPSystemEvent_NTPSystemEvent_ARRAYSIZE
@ NTPStatus_SyncSource_SYNC_TELEPHONE
@ NTPStatus_SyncSource_SYNC_UNKNOWN